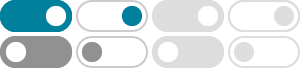
Hierarchical Inheritance with Examples in Python
Feb 13, 2024 · This Python code showcases hierarchical inheritance with a base class `Animal` and two derived classes, `Dog` and `Cat`. The `Animal` class initializes a `name` attribute and has a placeholder method `speak`.
A very easy tutorial to learn Python Objected-Oriented …
Dec 15, 2020 · In our example, we can define a class ‘Animal’ to store the information for cat and dog. class Animal: pass cat = Animal() dog = Animal() Python class name uses CapitalizedWords name convention. So if the class name is wild animal, the name is WildAnimal. Define Methods. class includes methods to describe the instance’s properties and ...
Python OOPs: Class, Object, Inheritance and Constructor with Example
Apr 3, 2019 · In this example, we have created a basic Animal class with an __init__ method (constructor) to initialize the object’s attributes (name and species). The make_sound method is a placeholder that can be overridden by subclasses.
Inheritance and Composition: A Python OOP Guide
Jan 11, 2025 · Say you have the base class Animal, and you derive from it to create a Horse class. The inheritance relationship states that Horse is an Animal. This means that Horse inherits the interface and implementation of Animal, and you can use Horse objects to replace Animal objects in the application.
python-class-tutorial/examples/2_2_animal_class.py at master - GitHub
class Animal: def __init__ (self, name, age): self.name = name self.age = age def __str__ (self): return f" {self.name} is {self.age} years old." def walk (self): return f" {self.name} starts walking." def jump (self): return f" {self.name} starts jumping."
Object-Oriented Programming (OOP) in Python – datanovia
Explore the fundamentals of Object-Oriented Programming in Python. This tutorial covers how to design classes, create objects, and implement inheritance and polymorphism to build modular and reusable code.
Object Oriented Programming in Python
Learn how Python implements object-oriented programming with classes, inheritance, encapsulation, polymorphism, and abstraction with practical examples. ... As you can see from the examples, Python’s implementation of OOP is flexible and intuitive, with less rigid syntax than languages like Java or C++.
Python OOP: Usage, and Examples - mimo.org
Object-oriented programming (OOP) in Python is a programming paradigm that organizes code into reusable objects. Python supports OOP principles such as encapsulation, inheritance, and polymorphism, making it an efficient language for structuring complex applications.
OOP in Python. Hello and welcome! Object-oriented… | by
Apr 21, 2023 · In this article, we’ll explore the basics of OOP in Python, including encapsulation, inheritance, polymorphism, abstraction, and best practices. Whether you’re a seasoned Python developer or...
Object Orientated Programming - Programming for Engineers
Define a class Animal with attributes: name, species, and diet. Add an __init__ method and a describe() method to describe the animal. Add a main() function at the bottom to create an instance of Animal and call describe(). Create a Python file named zoo_module.py. Define two classes, Animal and Zoo, within this file.
- Some results have been removed