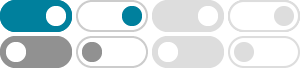
Implementation of 12 Sorting Algorithms in C++ - GitHub
You can find the implementation of Selection Sort, Insertion Sort, Binary-Insertion Sort, Bubble Sort, Shaker Sort, Shell Sort, Heap Sort, Merge Sort, Quick Sort, Counting Sort, Radix Sort, and Flash Sort in the sorting-methods folder.
GitHub - bornalgo/cpp-sorting-demos: A collection of well …
Explore various C++ sorting algorithms, including bubble, counting, insertion, merge, quick, radix, selection, and shell sorts. This repository contains well-documented code examples for each sorting algorithm, making it easy to understand and implement these essential algorithms in …
Sorting Algorithms - GeeksforGeeks
Apr 14, 2025 · A Sorting Algorithm is used to rearrange a given array or list of elements in an order. For example, a given array [10, 20, 5, 2] becomes [2, 5, 10, 20] after sorting in increasing order and becomes [20, 10, 5, 2] after sorting in decreasing order.
Writing a simple sorting algorithm in c++, along with a pseudo code …
Oct 31, 2012 · I'm looking to create an algorithm that takes an unsorted array,along with two integers (Lets say B AND C) and outputs TRUE, if A contains an element which is both greater than B and less than C, otherwise it returns FALSE. Count <-- j+1. while Count =< Length[A]
Sort in C++ Standard Template Library (STL) - GeeksforGeeks
Jan 11, 2025 · In this article, we will discuss various ways of sorting in C++. C++ provides a built-in function in C++ STL called sort () as the part of <algorithm> library for sorting the containers such as arrays, vectors, deque, etc. where, first: The beginning of the range to be sorted. last: The end of the range to be sorted.
How to implement a natural sort algorithm in c++?
Mar 13, 2009 · Several natural sort implementations for C++ are available. A brief review: natural_sort<> - based on Boost.Regex. In my tests, it's roughly 20 times slower than other options. Martin Pool's natsort - written in C, but trivially usable from C++.
How to implement classic sorting algorithms in modern C++?
The std::sort algorithm (and its cousins std::partial_sort and std::nth_element) from the C++ Standard Library is in most implementations a complicated and hybrid amalgamation of more elementary sorting algorithms, such as selection sort, insertion sort, quick sort, merge sort, or …
sorting-algorithm · GitHub Topics · GitHub
Mar 4, 2022 · Fast O(n) stable sorting algorithm. C++ implementation. It outperforms std::sort and std::stable_sort on N > 100 for both primitive types and complex objects.
Simple Sorting Algorithms with C++ | CodeSignal Learn
This lesson provides an overview and practical implementation of basic sorting algorithms in C++. It covers Bubble Sort, Selection Sort, and Insertion Sort, and also delves into the more advanced QuickSort algorithm.
Sorting in Data Structure: Beginners Guide with C++ Examples
Jan 28, 2025 · Sorting is a cornerstone of data structures and algorithms, and mastering it is essential for any programmer. In this article, we covered four popular sorting algorithms: Bubble Sort, Selection Sort, Insertion Sort, and Merge Sort, along with their C++ implementations.
- Some results have been removed