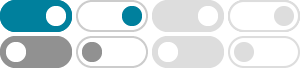
Implement a stack using singly linked list - GeeksforGeeks
Mar 20, 2025 · In this approach, we use a singly linked list, where each node contains data and a reference (or link) to the next node. To manage the stack, we maintain a top pointer that always points to the most recent (topmost) node in the stack. The key stack operations—push, pop, and peek can be performed using this top pointer.
Stack Using Linked List in C - GeeksforGeeks
May 8, 2024 · A linked list is a linear data structure used for storing a sequence of elements, where each element is stored in a node that contains both the element and a pointer to the next node in the sequence. Types of linked lists: Linked lists can be classified in the following categories Singly Linked List
Stack implementation using linked list, push, pop and display in C
Nov 8, 2015 · Before we perform any operation on stack, we must define its node structure. // Stack node structure struct stack { int data; struct stack *next; } *top; // Will contain size of stack int size = 0; How to push elements in stack using linked list. Insertion of new element to stack is known as push operation in stack. We can push elements at top ...
Stack Implementation using Linked List - Dot Net Tutorials
As you know, a linked list is a collection of nodes that hold a list or collection of elements. A stack is also a collection of elements. In a stack, insertion and deletion are done using the LIFO (Last In, First Out) discipline. Let us show you how the stack can be implemented using a linked list. Here, we have the basic structure of a linked ...
Data Structure - C Program to Implement Stack using Linked-List
Steps to build a linked-list based stack is as follows. Step 1: Declare pointers to build initial stack. struct node *head, *temp; The *head pointer of type struct points to the first node of the linked-list and the *temp pointer of type struct points to any new node you create. The pointers are created but does not point to anything.
Data Structures Tutorials - Stack Using Linked List with an …
We can use the following steps to insert a new node into the stack... Step 1 - Create a newNode with given value. Step 3 - If it is Empty, then set newNode → next = NULL. Step 4 - If it is Not Empty, then set newNode → next = top. Step 5 - Finally, set top = newNode. We can use the following steps to delete a node from the stack...
Stack using linked list - Codewhoop
Push (insert element in stack) The push operation would be similar to inserting a node at starting of the linked list; So initially when the Stack (Linked List) is empty, the top pointer will be NULL. Let's suppose we have to insert the values 1, 2 & 3 in the stack. So firstly we will create a new Node using the new operator and return its ...
Stack using Linked List | Data Structure Tutorial - Studytonight
Inserting Data in Stack (Linked List) In order to insert an element into the stack, we will create a node and place it in front of the list.
Stack Implementation using a Linked List – C, Java, and Python
Sep 14, 2022 · We can easily implement a stack through a linked list. In linked list implementation, a stack is a pointer to the “head” of the list where pushing and popping items happens, with perhaps a counter to keep track of the list’s size.
Stack Using Linked List in C: Implementation and Operations
In a linked list-based stack, each stack element is represented by a node, which contains two parts: data (the value stored in the node) and next (a pointer to the next node in the stack). The top pointer refers to the topmost node in the stack. Both push () and pop () operations occur at the top of the linked list, and they run in O (1) time.
- Some results have been removed