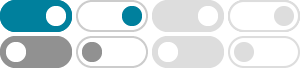
How do you assign all elements of an array at once?
When you initialize an array, you can assign multiple values to it in one spot: int array [] = {1,3,34,5,6}; ... but what if the array is already initialized and I want to completely replace the values of the elements in that array in one line:
c++ - A method to assign the same value to all elements in an array …
Dec 7, 2011 · int array[50]; std::fill(array, array + 50, number); If the number you want to set all the elements to is 0, you can do this shortcut: int array[50] = { }; Or if you're talking about std::vector, there is a constructor that takes the initial size of the vector and what to set each element to:
C++ Arrays - GeeksforGeeks
Apr 25, 2025 · To change the element at a particular index in an array, just use the = assignment operator with new value as right hand expression while accessing the array element. Example: {...} Traversing means visiting each element one by one.
How to set all elements of an array to zero or any same value?
May 7, 2014 · You must explicitly initialize all elements of the array using the initializer list. // initialize all elements to 4 int arr[4] = {4, 4, 4, 4}; // equivalent to int arr[] = {4, 4, 4, 4};
Different ways to Initialize all members of an array to the same value …
Jan 10, 2025 · Below are some of the different ways in which all elements of an array can be initialized to the same value: Initializer List: To initialize an array in C with the same value, the naive way is to provide an initializer list. We use this with small arrays. int num[5] = {1, 1, 1, 1, 1}; This will initialize the num array with value 1 at all index.
Arrays in C++ | Declare | Initialize | Pointer to Array Examples
Aug 10, 2024 · Instead of declaring each variable and assigning it a value individually, you can declare one variable (the array) and add the values of the various variables to it. Each value-added to the array is identified by an index.
How to Initialize an Array in C++? - GeeksforGeeks
Mar 18, 2024 · To initialize an array in C++, we can use the assignment operator = to assign values to the array at the time of declaration. The values are provided in a comma-separated list enclosed in curly braces {}.
C++ Array Assignment - CopyAssignment
Aug 31, 2022 · You can create different datatypes of arrays in C++ e.g. string, int, etc are the two most common types of C++ arrays that are commonly used. Today, we will see how to initialize and assign values to C++ arrays.
How to assign values to arrays in the C language?
In the C language, there are two methods to assign values to an array: Assign values one by one: assigning values to each element of the array through a loop. int arr[5]; int i; for (i = 0; i < 5; i++) { arr[i] = i + 1; for (i = 0; i < 5; i++) { printf("%d ", arr[i]); return 0; The output is:
Assign multiple values to array in C - Stack Overflow
You call GEN_ARRAY_ASSIGN_FUNC for every type and you add the type to the _Generic arguments. And use it like this: ARRAY_ASSIGN(coordinates, 1.f, 2.f, 3.f, 4.f);