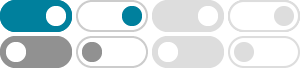
How do I generate random integers within a specific range in Java ...
For example if you want to generate five random integers (or a single one) in the range [0, 10], just do: Random r = new Random(); int[] fiveRandomNumbers = r.ints(5, 0, 11).toArray(); int randomNumber = r.ints(1, 0, 11).findFirst().getAsInt();
Generating Random Numbers in a Range in Java - Baeldung
May 11, 2024 · Let’s use the Math.random method to generate a random number in a given range [min, max): return (int) ((Math.random() * (max - min)) + min); Why does that work? Let’s look at what happens when Math.random returns 0.0, which is the lowest possible output: So, the lowest number we can get is min.
Generating Random Numbers in Java - GeeksforGeeks
Apr 24, 2025 · Generating Numbers in a Specific Range. The formula to generate a random number with a specific range is listed below: Random rand = new Random (); int randomNum = rand.nextInt (max – min + 1) + min; Note: min and max are our lower and higher limit of number. Example: 2. Using Math.random ()
Getting random numbers in Java - Stack Overflow
May 5, 2011 · The first solution is to use the java.util.Random class: import java.util.Random; Random rand = new Random(); // Obtain a number between [0 - 49]. int n = rand.nextInt(50); // Add 1 to the result to get a number from the required range // (i.e., [1 - 50]). n += 1; Another solution is using Math.random(): double random = Math.random() * 49 + 1 ...
How do I generate random integers within a specific range in Java ...
Dec 27, 2019 · Given two numbers Min and Max, the task is to generate a random integer within this specific range in Java. Examples: Output: 89. Input: Min = 100, Max = 899. Output: 514. Approach: Get the Min and Max which are the specified range.
Java: Generate Random Integers in Range - Stack Abuse
Feb 28, 2023 · To generate a single random integer, you can simply tweak the first argument of the ints() method, or use the findFirst() and getAsInt() methods to extract it from the IntStream: This results in a random integer in the range between 1..10 (second argument is exclusive):
Java - Generate random integers in a range - Mkyong.com
Aug 19, 2015 · In this article, we will show you three ways to generate random integers in a range. This Random().nextInt(int bound) generates a random integer from 0 (inclusive) to bound (exclusive). 1.1 Code snippet. For getRandomNumberInRange(5, 10), this will generates a random integer between 5 (inclusive) and 10 (inclusive). if (min >= max) {
Java 8 – Generate Random Number in Range - HowToDoInJava
Sep 6, 2023 · Learn to generate random numbers (integer, float, long or double) in a specified range (origin and bound) using new methods added in Java 8 in Random, SecureRandom and ThreadLocalRandom classes. private final static Random RANDOM = new Random(); Integer r1 = RANDOM.nextInt(0, 100); //A random number between 0 and 99 Float r2 = RANDOM.nextFloat ...
How do I generating random integers within a specific range in Java ...
Mar 10, 2025 · In this post, you will learn all about generating random integers in Java, particularly within a specified range. You will explore the Random class, discover how its methods work, and gain confidence in using them in your Java projects.
Generate random integers between specified ranges in Java
Mar 27, 2024 · This post will discuss how to generate random integers between the specified range in Java. 1. Using Random Class. We can use Random.nextInt() method that returns a pseudorandomly generated int value between 0 (inclusive) and the specified value (exclusive).
- Some results have been removed