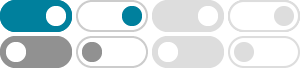
Python Program to Print all Odd Numbers in a Range
Nov 25, 2024 · There are several ways to print odd numbers in a given range in Python. Let’s look at different methods from the simplest to the more advanced. Using for loop with if condition. In this method, we iterate through all numbers in the range and check if each number is odd using the condition num%2! = 0. If true, the number is printed. Python
How to print odd numbers in python - Stack Overflow
Feb 6, 2022 · def print_odd_numbers(numbers): odd_numbers = list(filter(lambda x: x % 2 == 1, numbers)) for number in odd_numbers: print('Odd number:', number) nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11] print_odd_numbers(nums)
Python Program to Check if a Number is Odd or Even
Write a function to check if the entered integer is odd or even. If the given number is odd, return "Odd". If the given number is even, return "Even". For example, for input 4, the output should be "Even". Did you find this article helpful?
Check if a number is odd or even in Python - Stack Overflow
Similarly to other languages, the fastest "modulo 2" (odd/even) operation is done using the bitwise and operator: return 'odd' return 'even' The idea is to check whether the last bit of the number is set or not. If last bit is set then the number is odd, otherwise even.
Print Odd Numbers from 1 to 100 in Python – allinpython.com
write a program to print odd numbers from 1 to 100 in Python using for-loop, while-loop, function, etc. with proper algorithm and explanation.
Print odd numbers in a List - Python - GeeksforGeeks
Apr 14, 2025 · The most basic way to print odd numbers in a list is to use a for loop with if conditional check to identify odd numbers. Explanation: Loops through list a. For each element i, checks if i % 2 != 0 (i.e., if the number is odd). If the condition is true then print the odd number.
python - How do I determine if the user input is odd or even?
Dec 4, 2013 · It should have an output that looks something like this: The array contains odd and even numbers. The array contains only odd numbers. The array contains only even numbers. Here's what I have so far: numEnter = 5. numbers = [0] * numEnter. for index in range(numEnter): numbers[index] = int(input('Enter the numbers: ')) print(numbers)
How to Print Odd Numbers in Python using Range - Know …
How to Print Odd Numbers in Python using Range | The problem is to print the odd numbers in the given range. We can do this by three methods and also by using the range () method available in Python. The limits of range you can take from the end-user or …
How to Check If a Number Is Odd in Python | LabEx
Run the script again and enter 7.5: python odd_numbers.py. You should see the following output: Invalid input. Please enter an integer. Run the script again and enter 11: python odd_numbers.py Enter an integer: 11 11 is an odd number. This demonstrates how to validate the input type and handle potential errors using a try-except block. This is ...
5 Best Ways to Print Odd Numbers from a List in Python
Mar 11, 2024 · Problem Formulation: In this article, we will explore multiple ways to find and print odd numbers from a given list in Python. For example, given the input list [1, 2, 3, 4, 5], the desired output would be [1, 3, 5], isolating and printing only the odd numbers from the list.
- Some results have been removed