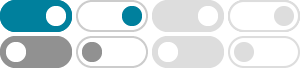
Create a Custom Exception in Java - Baeldung
May 11, 2024 · In this tutorial, we’ll cover how to create a custom exception in Java. We’ll show how user-defined exceptions are implemented and used for both checked and unchecked exceptions. Learn the basics of exception handling in Java as well as some best and worst practices. Read more →.
User-Defined Custom Exception in Java - GeeksforGeeks
Apr 16, 2025 · Create a User-Defined Custom Exception. Create a new class that extends Exception (for checked exceptions) or RuntimeException (for unchecked exceptions). Provide constructors to initialize the exception with custom messages. Add methods to provide additional details about the exception. (this is optional)
How to create a custom exception type in Java? [duplicate]
You should be able to create a custom exception class that extends the Exception class, for example: // Parameterless Constructor. public WordContainsException() {} // Constructor that accepts a message. public WordContainsException(String message) super(message); Usage: if(word.contains(" ")) throw new WordContainsException();
How to define custom exception class in Java, the easiest way?
Sep 23, 2010 · Typically you use super(message) in your constructor to invoke your parent constructor. For example, like this: public MyException(String message) { super(message); @vulkanino: No. The default constructor is added by the compiler for every class that defines no constructor of its own.
How to create custom exceptions in Java - CodeJava.net
Jul 9, 2019 · This Java tutorial guides you on how to create your own exceptions in Java. In the article Getting Started with Exception Handling in Java , you know how to catch throw and catch exceptions which are defined by JDK such as IllegalArgumentException , IOException , NumberFormatException , etc.
How to Create Custom Exception in Java - Java Guides
Creating custom exceptions in Java allows you to define your own exception types for specific error conditions. This can help make your code more readable and maintainable by providing meaningful exception types that are relevant to your application's logic.
Creating Exception Classes (The Java™ Tutorials - Oracle
When faced with choosing the type of exception to throw, you can either use one written by someone else — the Java platform provides a lot of exception classes you can use — or you can write one of your own.
Java Custom Exception - Online Tutorials Library
To create a custom exception, you need to create a class that must be inherited from the Exception class. Here is the syntax to create a custom class in Java - You just need to extend the predefined Exception class to create your own Exception. These are considered to …
Java Program to Create custom exception
In the above example, we have extended the Exception class to create a custom exception named CustomException. Here, we call the constructor of Exception class from the CustomException class using super keyword.
How to Create Your Own Exception in Java Step-by-Step
Jan 11, 2024 · Follow these essential steps. Extend an Exception Class. For an unchecked exception, extend the RuntimeException class. Example Implementation: InvalidAgeException(String s) { super(s); In this example, the InvalidAgeException extends the Exception class, creating a checked custom exception.