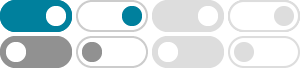
How do I declare and initialize an array in Java?
Jul 29, 2009 · Static Array: Fixed size array (its size should be declared at the start and can not be changed later) Dynamic Array: No size limit is considered for this. (Pure dynamic arrays do not exist in Java. Instead, List is most encouraged.) To declare a static array of Integer, string, float, etc., use the below declaration and initialization statements.
java - Convert an integer to an array of digits - Stack Overflow
I try to convert an integer to an array. For example, 1234 to int[] arr = {1,2,3,4};. I've written a function: public static void convertInt2Array(int guess) { String temp = Integer.toString(g...
add an element to int [] array in java - Stack Overflow
Apr 9, 2013 · The size of an array can't be changed. If you want a bigger array you have to create a new array. However, a better solution would be to use an (Array)List which can grow as you need it. The method ArrayList.toArray(T[] a) returns …
java - int[] and Integer[] arrays - What is the difference? - Stack ...
Sep 17, 2013 · Integer is an object. When you have an array of Integers, you actually have an array of objects. Array of ints is an array of primitive types. Since arrays are objects, they're allocated on the heap. If it's an array of ints, these ints will be allocated on the heap too, within the array. You may find this link helpful.
What's the simplest way to print a Java array? - Stack Overflow
Yes ! this is to be mention that converting an array to an object array OR to use the Object's array is costly and may slow the execution. it happens by the nature of java called autoboxing. So only for printing purpose, It should not be used. we can make a function which takes an array as parameter and prints the desired format as
Convert String to int array in java - Stack Overflow
Feb 27, 2022 · substring removes the brackets, split separates the array elements, trim removes any whitespace around the number, parseInt parses each number, and we dump the result in an array. I've included trim to make this the inverse of Arrays.toString(int[]) , but this will also parse strings without whitespace, as in the question.
Java, Simplified check if int array contains int - Stack Overflow
Aug 18, 2012 · Find an integer in an Array in a short manner in Java. 0. Checking if an array contains certain integers. 0.
How to convert int [] into List<Integer> in Java? - Stack Overflow
Jul 2, 2009 · Arrays.asList will not work as some of the other answers expect. This code will not create a list of 10 integers.
Convert an integer to an array of characters : java
What is the best way to convert an integer into a character array? Input: 1234 Output: {1,2,3,4} Keeping in mind the vastness of Java language what will be the best and most efficient way of doi...
What is the default initialization of an array in Java?
Java says that the default length of a JAVA array at the time of initialization will be 10. private static final int DEFAULT_CAPACITY = 10; But the size() method returns the number of inserted elements in the array, and since at the time of initialization, if you have not inserted any element in the array, it will return zero.