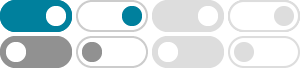
Program to check if an Array is Palindrome or not
Jan 20, 2023 · Given an array, the task is to determine whether an array is a palindrome or not. Examples: Input: arr[] = {3, 6, 0, 6, 3} Output: Palindrome Input: arr[] = {1, 2, 3, 4, 5} Output: Not Palindrome . Approach: Initialise flag to unset int flag = 0. Loop the array till size n/2; In a loop check if arr[i]! = arr[n-i-1] then set the flag = 1 and break
java - Check if an array is palindrome - Stack Overflow
Mar 20, 2019 · You could check if the array is palindrome by comparing it with reversed copy of the original array. Using ArrayUtils.reverse from Apache commons: int[] arrCopy = Arrays.copyOf(array, array.length); ArrayUtils.reverse(arrCopy); boolean isPalindrome = Arrays.equals(array, arrCopy);
Check if all elements of the array are palindrome or not
Dec 30, 2018 · Given an array arr[]. The task is to check if the array is PalinArray or not i.e., if all elements of array are palindrome or not. Examples: Input: arr[] = [21, 131, 20] Output: false Explanation: For the given array, element 20 is not a palindrome so false is the output. Input: arr[] = [111, 121, 222, 333, 444] Output: true
2 ways to check for palindrome array in java - codippa
March 27, 2020 - Learn what is palindrome array and how to check if an array is palindrome in java in 2 different ways with example programs and explanation.
Check Array Palindrome using Java - Daily Java Concept
Jan 23, 2024 · This program provides an efficient solution to check if an array is a palindrome. Leveraging pointers and iterative comparison, the method ensures accurate validation of palindromic arrays. Incorporate this approach into your Java projects to unravel the mysteries of array palindromes. Happy coding! Latest Posts: Merging Two Sorted Arrays in Java
java - Finding Palindromes in an Array - Stack Overflow
May 29, 2014 · Write a method isPalindrome that accepts an array of Strings as its argument and returns true if that array is a palindrome (if it reads the same forwards as backwards) and /false if not. For example, the array {"alpha", "beta", "gamma", "delta", "gamma", "beta", "alpha"} is a palindrome, so passing that array to your method would return true.
java - Checking Palindromes using an array - Stack Overflow
Feb 22, 2017 · You can use this method also to check if the string is palindrome or not. public static boolean pallindrom(String S) { char arr[] = S.toCharArray(); int flag = 0; for(int i = 0 ; i < (arr.length+1)/2 ; i++) { if(arr[i] != arr[arr.length-1-i]) { flag = 1; break; } } if(flag == 0) return true; else return false; }
Palindrome Program In Java – 5 Ways | Programs - Java Tutoring
Apr 17, 2025 · Palindrome program in Java – Here, we will discuss the various methods to check whether a given number is a palindrome or not. A compiler is added so that you can execute the program yourself, alongside various examples and sample outputs are given.
Check if rearranging Array elements can form a Palindrome or …
Aug 25, 2021 · Given a positive integer array arr of size N, the task is to check if number formed, from any arrangement of array elements, form a palindrome or not. Examples: Input: arr = [1, 2, 3, 1, 2]
java - Trying to solve a palindrome using integer arrays - Stack Overflow
Mar 11, 2015 · First you're not checking if a number is a palindrome or not. Your algorithm is flawed; Second, you're asking to enter a size but in the end, the user inputs it but you don't use it yourself. Instead, you're using that introduced value in …
- Some results have been removed