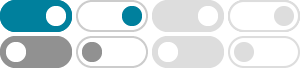
Python Program to Implement Stack Using Linked List
Apr 8, 2024 · In Python, creating a stack using a linked list involves implementing a data structure where elements are added and removed in a last-in-first-out (LIFO) manner. This approach uses the concept of nodes interconnected by pointers, allowing efficient insertion and …
Implement a stack using singly linked list - GeeksforGeeks
Mar 20, 2025 · Easy implementation: Implementing a stack using a singly linked list is straightforward and can be done using just a few lines of code. Versatile : Singly linked lists can be used to implement other data structures such as queues, linked lists, and trees.
Implementing a Stack Using a Linked List in Python: Top 5 …
Mar 7, 2024 · This article illustrates how to implement a stack using a linked list in Python, ensuring efficient O(1) time complexity for push and pop operations. We will start with an empty stack and show how elements can be pushed onto the …
Python | Stack using Doubly Linked List - GeeksforGeeks
Jan 5, 2023 · 1. push () : Insert the element into Stack and assign the top pointer to the element. 2. pop () : Return top element from the Stack and move the top pointer to the second element of the Stack. 3. top () : Return the top element. 4. size () : Return the Size of the Stack. 5. isEmpty () : Return True if Stack is Empty else return False.
Python Program to Implement Stack using Linked List
This is a Python program to implement a stack using a linked list. The program creates a stack and allows the user to perform push and pop operations on it. 1. Create a class Node with instance variables data and next. 2. Create a class Stack with instance variable head. 3. The variable head points to the first element in the linked list. 4.
Implementing Stack in Python using Linked List - HackerNoon
Feb 5, 2022 · In this tutorial we have implemented a stack using linked list and concepts of OOP in Python programming language. A stack is a LIFO data structure in which items can be added and removed, both from the same point. A linked list is a data structure made up of small nodes each of which can store a data item and an address to the next such node.
Stack using Linked List in Python - PrepInsta
To implement a Stack using Linked List in Python, you can modify the linked list code from the previous response. In a stack, elements are added and removed from the top, following the Last-In-First-Out (LIFO) principle. In this page, we will delve deep into the implementation of stacks using linked list in python, offering you a clear and ...
Stack Implementation using a Linked List – C, Java, and Python
Sep 14, 2022 · We can easily implement a stack through a linked list. In linked list implementation, a stack is a pointer to the “head” of the list where pushing and popping items happens, with perhaps a counter to keep track of the list’s size.
Python Program to Implement a Stack Using Linked List
Aug 29, 2024 · The following is a description of an algorithm to implement a stack using a linked list: Define a class for the node of the linked list. Each node should contain the data (the item being added to the stack) and a pointer to the next node in the stack.
Stack Implementation Using Linked List in Python
Sep 18, 2024 · This program demonstrates the implementation of a stack data structure using a linked list. Stacks are a type of data structure with Last In First Out (LIFO) access policy. The operations demonstrated in this stack are: Push: Add an item to the top of the stack. Pop: Remove the item at the top of the stack.
- Some results have been removed