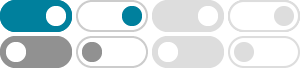
python - Conditional traffic light system - Code Review Stack …
Oct 28, 2020 · I have written Python code which uses multiple if conditions and a for loop. The main objective of the code is to produce a traffic light system based on certain conditions. It takes 4 months (m0, m1, m2, m3) and dataframe as an input, runs the condition through each row, and returns -1, 0 or 1.
Traffic light program using while loop in python - Stack Overflow
Jan 11, 2018 · while True: if stop_light >= 1 and stop_light < 10: print('Green light') stop_light += 1 elif stop_light < 20: print('Yellow light') stop_light += 1 elif stop_light < 30: print("Red light") stop_light += 1 else: stop_light = 0 if stop_light == 0: break
Traffic signal Program in Python [4 Approaches] - Python Guides
Feb 12, 2024 · Here is an example of using the generator for a traffic signal program in Python: Code. import time def traffic_signal(): colors = ["Red", "Green", "Yellow"] durations = [5, 5, 2] while True: for color, duration in zip(colors, durations): yield color, duration def display(color): print(f"{color} light - {'Stop' if color == 'Red' else 'Go' if ...
Python-Conditional-Statements/TrafficLightSimulator.py at main …
traffic_light = str(input("Please enter a traffic light color: Red, Yellow, or Green: ").lower()) if traffic_light == "red": print("Please stop the vehicle, immediately or I am calling the cops!!!")
python 3.x - Traffic light while loop - Stack Overflow
Oct 26, 2017 · The code. elif stop_light < 30: print ("Red") stop_light += 1 will only run up to and including when stop_light equals 29 and adding 1 on 29 will turn it to 30 and so it will never be 31. To fix this you need to change the < to <=, which means less than or equal to, or change 30 to 31.
Learn Conditional Statements in Python With Turtle - The Turing …
Jan 27, 2024 · In this function, we use an if statement followed by two elif statements and an else statement. The traffic light changes to green, yellow, or red depending on the input. If the input is anything other than these colors, it prints "Invalid color." Interactive Control with Keyboard Events
Python code for Simulating a Traffic Light - Example Project
Aug 17, 2023 · This Python code simulates a traffic light using two user-defined functions. The first function, trafficLight(), prompts the user to enter the color of the traffic light and then calls the second function, light(), to determine the corresponding message.
4. Control Statements — Python, No Tears 1.0.0 documentation
The most basic form of if/else statements comes when you are only using if. Below, you have the state of the traffic light (which is yellow), and you want to test to see if the traffic light is yellow. If the traffic light is yellow, you print slow down.
Building a Traffic Simulation System in Python: A Step-by-Step …
Aug 19, 2024 · In this tutorial, we’ll walk through building a traffic simulation system using Python, organized with a Domain-Driven Design (DDD) structure. Domain-Driven Design is a software …
Simulate a car at a traffic signal using IF-ELIF-ELSE in Python.
Mar 12, 2022 · Simulate a car at a traffic signal using IF-ELIF-ELSE in Python. Accept the colour of the traffic light from the user and display whether the car should stop, slow down or move based on the colour given.