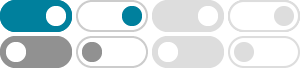
Binary Tree in Python - GeeksforGeeks
Feb 27, 2025 · A Binary search tree is a binary tree where the values of the left sub-tree are less than the root node and the values of the right sub-tree are greater than the value of the root node. In this article, we will discuss the binary search tree in Python.
python - How to implement a binary tree? - Stack Overflow
Feb 1, 2017 · Here is my simple recursive implementation of binary search tree. def __init__(self, val): self.l = None. self.r = None. self.v = val. def __init__(self): self.root = None. def get_root(self): return self.root. def add(self, val): if not self.root: self.root = Node(val) else: self._add(val, self.root) def _add(self, val, node): if val < node.v:
Binarytree Module in Python - GeeksforGeeks
Jan 10, 2023 · The binary search tree is a special type of tree data structure whose inorder gives a sorted list of nodes or vertices. In Python, we can directly create a BST object using binarytree module. bst() generates a random binary search tree and return its root node. Syntax: binarytree.bst(height=3, is_perfect=False) Parameters:
How can I implement a tree in Python? - Stack Overflow
Mar 1, 2010 · Each n-ary tree can be represented by binary tree. I recommend anytree (I am the author). Example: print("%s%s" % (pre, node.name)) ├── Jet. ├── Jan. └── Joe. anytree has also a powerful API with: walking from one node to an other.
Python Binary Tree - Online Tutorials Library
Python Binary Tree - Learn about Python binary trees, their properties, types, and implementation details. Explore how to create and manipulate binary tree structures in Python.
Binary Trees in Python: Implementation and Examples
Jun 19, 2024 · Nodes: In a binary tree, a node represents a single element of the tree. Each node contains a value or data, and pointers (or references) to its left and right children, if they exist. Edges: Edges are the connections between nodes. An edge connects a parent node to a child node. Root: The root is the topmost node in a binary tree. It is the ...
Binary Tree implementation in Python - AskPython
Feb 10, 2021 · In this tutorial, we will learn about what binary trees are and we will study underlying concepts behind binary tree data structure. We will also implement them using classes in python. What is a Binary Tree? A Binary tree is a data structure in which there is a parent object and each object can have zero, one or two children.
Tree Data Structure in Python - PythonForBeginners.com
Jun 9, 2023 · We can define a Tree node of the structure shown above in Python using classes as follows. Here, the constructor of the Tree node takes the data value as input, creates an object of BinaryTreeNode type and initializes the data field equal to the given input, and initializes the references to the children to None.
Binary Tree Implementation in Python: A Comprehensive Guide
Apr 8, 2025 · In Python, implementing a binary tree allows for efficient manipulation and traversal of hierarchical data. This blog will walk you through the concepts, usage, common practices, and best practices of binary tree implementation in Python.
Binary Trees in Python: A Comprehensive Guide - CodeRivers
Mar 17, 2025 · In Python, working with binary trees can be both efficient and elegant. This blog will explore the basic concepts of binary trees, how to implement them in Python, common operations, and best practices. A binary tree is a tree - like data structure where each node has at most two children.
- Some results have been removed