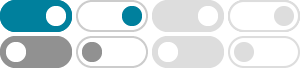
Python code for Round Robin Scheduling algorithm for CPU scheduling
Apr 8, 2021 · The code should do the following: Report the time each process is completed; Report wait times of each process in the queue; The #CODE indicates where the code is missing.
Program for Round Robin Scheduling for the Same Arrival Time
Jan 13, 2025 · Round Robin is a CPU scheduling algorithm where each process is cyclically assigned a fixed time slot. It is the preemptive version of the First come First Serve CPU Scheduling algorithm. This article focuses on implementing a Round Robin Scheduling Program where all processes have the same arrival time. In this scenario, all processes arrive ...
python - Round Robin using Queue - Stack Overflow
Jul 1, 2023 · def round_robin(processes, time_quantum): rr = Queue() for process in processes: rr.put(process) waiting_time = [0] * len(processes) turn_around_time = [0] * len(processes) curr_time = 0 while not rr.empty(): process = rr.get() if process.remaining_time <= time_quantum: curr_time += process.remaining_time process.completion_time = curr_time ...
Round Robin Scheduling Algorithm with Different Arrival Time
Jan 13, 2025 · In this article, we’ll explore how the Round Robin Scheduling Algorithm effectively handles this situation. Also, we’ll see its program implementation. Handles Dynamic Arrival: Processes can arrive at different times, and the scheduler dynamically adds them to the ready queue as they arrive, ensuring no process is overlooked.
GitHub - InfinityAbir/Round-Robin-Scheduling-Algorithm-: This is python …
This repository contains a Python implementation of the Round Robin Scheduling Algorithm, a CPU scheduling technique commonly used in operating systems. It ensures fair process execution by assigning each process a fixed time quantum and cycling through them in a …
How to create a round robin scheduling program in Python
May 4, 2023 · How Round Robin Scheduling Python Algorithm will work; Why use Python to create Round Robin Scheduling program; Using the Nylas Quickstart Guide; Getting Started with Round Robin Scheduling in Python – Step by Step; Step 2. Create a Calendar for Each User; Step 3: Allow user to modify a specific scheduler; Build for Free!!
Python/scheduling/round_robin.py at master - GitHub
Return: The waiting time for each process. >>> calculate_waiting_times ( [10, 5, 8]) [13, 10, 13] >>> calculate_waiting_times ( [4, 6, 3, 1]) [5, 8, 9, 6] >>> calculate_waiting_times ( [12, 2, 10]) [12, 2, 12] """ quantum = 2 rem_burst_times = list (burst_times) waiting_times = [0] * len (burst_times) t = 0 while True: done = True ...
A round-robin algorithm implementation written in Python. #round-robin …
# Switch sides after each round: if(i % 2 == 1): s = s + [ zip(l1, l2) ] else: s = s + [ zip(l2, l1) ] list.insert(1, list.pop()) return s: def main(): for round in create_schedule(div1): for match in round: print match[0] + " - " + match[1] print: for round in create_schedule(div2): for match in round: print match[0] + " - " + match[1] print
Round Robin Scheduling Algorithm in Python - Tpoint Tech
Jan 5, 2025 · In this article, we are learning about round robin scheduling algorithm in Python. Round-robin is a CPU scheduling algorithm in which each round is assigned a time slot in a round-robin process. It is a pre-emptive version of the first come, first served or FCFS CPU scheduling algorithm.
Python Round Robin Scheduling Algorithm - CodePal
In this Python code, we have implemented the Round Robin scheduling algorithm and provided a function that accepts arrival time as input and calculates the average waiting time and average turnaround time for the processes.
- Some results have been removed