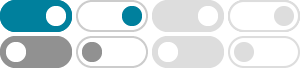
Creating Queue Using Collections.Deque In Python
Apr 24, 2024 · In Python, Queue and Deque are the data structures used for managing collections of elements in a first-in, first-out (FIFO) manner. In this article, we will learn to implement queues using collections.deque in Python.
Deque in Python - GeeksforGeeks
Mar 6, 2025 · A deque stands for Double-Ended Queue. It is a data structure that allows adding and removing elements from both ends efficiently. Unlike regular queues, which are typically operated on using FIFO (First In, First Out) principles, a deque supports both FIFO and LIFO (Last In, First Out) operations.
How to use deque in Python (collections.deque) | note.nkmk.me
Apr 20, 2025 · In Python, the collections.deque class provides an efficient way to handle data as a queue, stack, or deque (double-ended queue). While the built-in list can be used as a queue, stack, or deque, collections.deque offers better performance, especially for adding or removing elements at the beginning.
Deque Implementation in Python - GeeksforGeeks
Feb 13, 2025 · Deque in Python is implemented using a doubly linked list, making append and pop operations from both ends O (1) (constant time). Unlike Python’s built-in list (which uses a dynamic array), deque does not require shifting elements when inserting/deleting at the front. Different methods to implement Deque are: 1. Using Doubly Linked List.
Python type hinting a deque filled with myclass objects
Aug 21, 2018 · In Python 3.9, you can use deque['MyClass']() directly. If you are using Python 3.6.1 or higher, you can use typing.Deque: pass. return myqueue.popleft() global_queue.append(MyClass()) Alternatively, you can do global_queue = Deque['MyClass']() instead -- at runtime, that'll construct a collections.deque object.
Deque in Python using Collections Module
Jun 1, 2022 · In Python, we can use the collections.deque class to implement a deque. The deque class is a general-purpose, flexible and efficient sequence type that supports thread-safe, memory efficient appends and pops from either side. A deque provides approximately O (1) time complexity for append and pop opeations in either direction.
Understanding Python's deque - Python Central
Python's collections module provides a powerful data structure called deque (pronounced "deck"), which stands for "double-ended queue." A deque is a generalization of stacks and queues, allowing you to add or remove elements from both ends efficiently.
Deque in Python: A Comprehensive Guide to Efficient Data …
In this comprehensive guide, we’ll explore the deque data structure in Python, its features, use cases, and how it can improve your coding efficiency. Table of Contents. What is a Deque? Importing Deque in Python; Creating a Deque; Basic Operations with Deque; Advanced Operations and Methods; Deque vs. List: Performance Comparison; Common Use ...
How to Use deque for Efficient List Operations in Python
In Python, the collections.deque (double-ended queue) is a powerful data structure optimized for fast insertions and deletions from both ends. Unlike Python lists, which have an O (n) time complexity for insertions and deletions at the beginning, deque performs these operations in …
Using Deque in Python | Python Queues and Stacks Guide
Aug 13, 2023 · An example of using deque() in Python: from collections import deque # Create a deque queue = deque() # Enqueue elements queue.append('A') queue.append('B') queue.append('C') # Dequeue elements queue.popleft() # …
- Some results have been removed