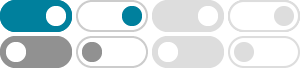
Components and Props – React - reactjs.org
Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen. Function and Class Components . The simplest way to define a component is to write a JavaScript function:
Passing Functions to Components – React - reactjs.org
How do I bind a function to a component instance? There are several ways to make sure functions have access to component attributes like this.props and this.state , depending on which syntax and build steps you are using.
Using the State Hook – React - reactjs.org
A Hook is a special function that lets you “hook into” React features. For example, useState is a Hook that lets you add React state to function components. We’ll learn other Hooks later. When would I use a Hook? If you write a function component and realize you need to add some state to it, previously you had to convert it to a class.
Typechecking With PropTypes – React - reactjs.org
Function Components . If you are using function components in your regular development, you may want to make some small changes to allow PropTypes to be properly applied. Let’s say you have a component like this:
React.Component – React - reactjs.org
React lets you define components as classes or functions. Components defined as classes currently provide more features which are described in detail on this page. To define a React component class, you need to extend React.Component:
Hooks at a Glance – React - reactjs.org
Hooks are functions that let you “hook into” React state and lifecycle features from function components. Hooks don’t work inside classes — they let you use React without classes. (We don’t recommend rewriting your existing components overnight but you can start using Hooks in the new ones if you’d like.)
Components 與 Props – React - reactjs.org
概念上來說,component 就像是 JavaScript 的 function,它接收任意的參數(稱之為「props」)並且回傳描述畫面的 React element。 Function Component 與 Class Component . 定義 component 最簡單的方法即是撰寫一個 Javascript function:
Composition vs Inheritance – React - reactjs.org
React has a powerful composition model, and we recommend using composition instead of inheritance to reuse code between components. In this section, we will consider a few problems where developers new to React often reach for inheritance, and show how we can solve them with composition.
Invalid Hook Call Warning – React - reactjs.org
You can only call Hooks while React is rendering a function component: Call them at the top level in the body of a function component. Call them at the top level in the body of a custom Hook. Learn more about this in the Rules of Hooks.
Context – React - reactjs.org
A React component that subscribes to context changes. Using this component lets you subscribe to a context within a function component. Requires a function as a child. The function receives the current context value and returns a React node.