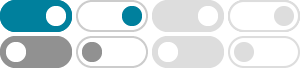
python - Find the division remainder of a number - Stack Overflow
How could I go about finding the division remainder of a number in Python? For example: If the number is 26 and divided number is 7, then the division remainder is 5. (since 7+7+7=21 and 26-21=5.)
Modulo operator (%) in Python - GeeksforGeeks
Feb 17, 2025 · Modulo operator (%) in Python gives the remainder when one number is divided by another. Python allows both integers and floats as operands, unlike some other languages. It follows the Euclidean division rule, meaning the remainder …
Python math.remainder() Method - W3Schools
# Return the remainder of x/y print (math.remainder(9, 2)) print (math.remainder(9, 3)) print (math.remainder(18, 4))
Python Program to find the Quotient and Remainder of two …
Jul 31, 2022 · Given two numbers n and m. The task is to find the quotient and remainder of two numbers by dividing n by m. Examples: Output: . Input . Output: . Method 1: Naive approach. The naive approach is to find the quotient using the double division (//) operator and remainder using the modulus (%) operator. Example: Output: Time Complexity: O (1)
modulo - Python remainder operator - Stack Overflow
Aug 29, 2013 · Because Python uses floored division (following Knuth's argument in The Art of Computer Programming), it uses the matching remainder operator. If you want either of the other, you have to write it manually. It's not very hard; this Wikipedia article shows how to implement all three. For example: q = a / b. q = -int(-q) if q<0 else int(q)
Python Modulo Operator: Examples, Uses and Importances
When dividing one integer by another, you can use the Python Modulo operator (“%”) to calculate the remainder. This operator returns an integer result and helps determine whether a number is even or odd, handling cyclic patterns and performing various mathematical tasks.
Python Modulo - Using the % Operator - Python Geeks
One possible way to check if a number is divisible by another number is by using the modulo operator. If the resulting remainder is 0, then the number is indeed divisible. Here is an example: You can also use the modulo operator for indexing.
How to Get Division Remainder in Python - Delft Stack
Mar 11, 2025 · This tutorial demonstrates how to get the remainder of the division in Python. Learn about the modulus operator, the divmod function, and alternative methods to effectively calculate division remainders.
Python Remainder: Concepts, Usage, and Best Practices
Jan 24, 2025 · In Python, the remainder operation is a fundamental arithmetic operation that provides valuable insights into the relationship between two numbers. Understanding how to calculate remainders is crucial for various programming tasks, such as divisibility checks, cyclic algorithms, and number theory applications.
Understanding the Remainder in Python: Concepts, Usage, and …
Feb 4, 2025 · Given two numbers a and b, a % b returns the remainder when a is divided by b. Mathematically, if a = b * q + r, where q is the quotient and r is the remainder, then a % b gives the value of r. The remainder r always satisfies the condition 0 <= r < b when a and b are non - negative integers.
- Some results have been removed