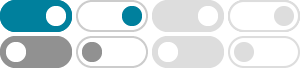
How can I find the number of elements in an array?
It is not possible to find the number of elements in an array unless it is a character array. Consider the below example: int arr[100]={1,2,3,4,5}; int size = sizeof(arr)/sizeof(arr[0]); printf("%d", size); return 1; The above value gives us value 100 even if the number of elements is five.
Element count of an array in C++ - Stack Overflow
Since C++17 you can also use the standardized free function: std::size(container) which will return the amount of elements in that container. example: Arrays in C++ are very different from those in Java in that they are completely unmanaged.
C++ Arrays - GeeksforGeeks
Apr 25, 2025 · The size of the array in bytes can be determined by the sizeof operator using which we can also find the number of elements in the array. We can find the size of the type of elements stored in an array by subtracting adjacent addresses.
How to Find the Length of an Array in C++? - GeeksforGeeks
Nov 29, 2024 · In C++, the length of an array is defined as the total number of elements present in the array. In this article, we will learn how to find the length of an array in C++. The simplest way to find the length of an array is by using the sizeof operator.
count() in C++ STL - GeeksforGeeks
Nov 27, 2024 · In C++, the count() is a built-in function used to find the number of occurrences of an element in the given range. This range can be any STL container or an array. In this article, we will learn about the count() function in C++. Let’s take a quick look at a simple example that uses count() method: C++
Get Number of Elements in Array in C++ - Java2Blog
Oct 8, 2021 · We will understand how to find the total number of elements of an array in this article. Get Number of Elements in Array in C++ Using the sizeof() function. The sizeof() function in C++ returns the size of the variable during compile time. Arrays store elements of …
How to Count number of elements in array in C++ - CodeSpeedy
Solution to "How to count number of elements in an array in C++" with user-entered elements and pre-entered elements with source code and explanation.
Find the count of elements in array in C++ - Stack Overflow
How do I find the number of elements filled in the array? I have tried. int size= sizeOf(arr)/sizeof(int); but this gives me 100. I only want to get the elements which I have allowed the user to enter. Actually, there is a situation where the number of generated outputs can be any number. So I assigned each value in a separate array as arr ...
calc. number of elements in array - C++ Forum - C++ Users
Aug 22, 2008 · The sizeof() function returns the number of bytes occupied by a variable or array. If you know that, then you just need to divide by the number of bytes that each element of the array occupies. int num_elements = sizeof( array ) / sizeof( array[0] );
9.4: Finding a Specific Member of an Array
Jun 18, 2021 · In C++, we can use sizeof operator to find number of elements in an array. Notice we have to calculate the size based on the entire size of memory taken up by the array divided by the sizeof one element of the array.
- Some results have been removed