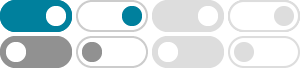
How do I add together integers in a list (sum a list of numbers) in python?
Dec 17, 2012 · First Way: my_list = [1,2,3,4,5] list_sum = sum(list) Second Way (less efficient): my_list = [1,2,3,4,5] list_sum = 0 for x in my_list: list_sum += x
Python's sum (): The Pythonic Way to Sum Values
In this step-by-step tutorial, you'll learn how to use Python's sum () function to add numeric values together. You also learn how to concatenate sequences, such as lists and tuples, using sum ().
python - Add to integers in a list - Stack Overflow
Jun 2, 2016 · Yes, it is possible since lists are mutable. Look at the built-in enumerate() function to get an idea how to iterate over the list and find each entry's index (which you can then use to …
Sum Of Elements In A List In Python - PythonForBeginners.com
Jan 9, 2022 · The first way to find the sum of elements in a list is to iterate through the list and add each element using a for loop. For this, we will first calculate the length of the list using the len () method.
How do I add/combine a list and a integer together into a list (Python …
Mar 20, 2017 · You can simply add the line: output.append(sumVal) to the program just before the return statement to add the total sum of the odd parts of the tuples, like: def sorting(tup1, tup2): output = [] sumVal = 0 wholeTup = tup1 + tup2 for i in range(0, len(wholeTup)): result = " " if i % 2 == 0 or i == 0: word = wholeTup[i].title() output.append(word)
5 Best Ways to Sum a List of Integers in Python - Finxter
Feb 24, 2024 · The most straightforward method to sum a list of integers in Python is by using the built-in sum() function. It takes an iterable as an argument and returns the sum of all its elements. The function is optimized and concise. Here’s an example: Output: 15. This code snippet demonstrates the simplicity of Python’s sum() function.
Add Integers to a List in Python - Stack Abuse
Jul 1, 2022 · In Python, you can add integers to a list using a variety of methods, a few of which we'll take a look at here. One of the most common ways to add an integer to a list, let alone any other type of data, is to use the append() method. >>> int_list.append(4) This will simply add a single integer to the very end of the list.
How to Add All Numbers in a List Python
Nov 20, 2023 · To add all numbers in a list, you can use the built-in function sum () in Python. Here is an example on how it works:
How to Add Numbers in a List Python
Jul 21, 2023 · Python provides a built-in function named sum() that allows you to add all elements within an iterable, such as a list, together. This function is particularly useful when dealing with arithmetic operations involving multiple values.
Summing Elements from a List in Python - CodeRivers
Feb 6, 2025 · Here, we use a list comprehension [my_list [i] for i in indices] to create a new list containing the elements at the specified indices, and then we use the sum () function to add them together. We can also sum elements in a list that meet a certain condition. For example, let's sum all the even numbers in a list.