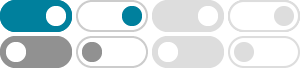
dictionary - How to use dot notation for dict in python ... - Stack ...
Apr 29, 2013 · Adding, modifying and removing values is achieved with regular attribute access, i.e. you can use statements like n.key = val and del n.key. To go back to a dict again: >>> vars(n) {'key1': 'value1', 'key2': 'value2'}
python - How to print the value of a specific key from a dictionary …
Apr 28, 2024 · If you want to check whether a key has been successfully added or not, use below command: print('key' in dictionary) for eg: print('New york' in USA)
How to use a dot "." to access members of dictionary?
Mar 1, 2010 · >>> obj = YourObject(key="value") >>> print(obj.key) "value" ... to convert it to a dict: >>> print(obj.__dict__) {"key": "value"}
Python Print Dictionary Keys and Values - GeeksforGeeks
Sep 27, 2024 · In this article, we'll explore different methods to Print Dictionary Keys and Values. Example: Using print () Method. Explanation: In this example, below Python code defines a dictionary `my_dict` with key-value pairs. It then prints the keys, values, and the entire dictionary.
Using dot "." notation to access dictionary keys in Python
Apr 9, 2024 · # Using dot "." notation to access nested dictionary keys. To use dot notation to access nested dictionary keys: Iterate over the dictionary's items in the __init__ method of a class. If the key has a value of type dict, instantiate the class …
Solved: How to Use Dot Notation for Dictionary Access in Python
Nov 6, 2024 · Let’s explore a scenario where you have a dictionary and you want to access its values using dot notation instead of the traditional bracket notation. Example Usage of Dictionary in Python test = dict() test[ 'name' ] = 'value' print(test[ 'name' ]) # Output: value
How to Print a Dictionary in Python - GeeksforGeeks
Sep 27, 2024 · Print a Dictionary in Python Using pprint Module. In this approach, we are importing the pprint module and using it to print the contents of the input_dict without using any looping. Using this module, we print the dicoanory in a more readable format as each of the key: value pair is printed in separate lines. Python
Using Dot Notation for Dictionaries in Python 3
Jan 17, 2024 · To access a value in a dictionary, you traditionally use square brackets and the key: print(person["name"]) # Output: John Using Dot Notation for Dictionary Access. In Python 3, you can also access values in dictionaries using dot notation. This syntax is similar to accessing attributes of an object in object-oriented programming languages.
Master the Python Dictionary Dot Notation for Easy
With dot notation, Python developers can access and manipulate nested dictionary values using a simple dot syntax, instead of using multiple bracket pairs for each level of nesting. How Dot Notation Works
Python Dictionary: Guide To Work With Dictionaries In Python
A Python dictionary is a collection of key-value pairs where each key must be unique. Think of it like a real-world dictionary where each word (key) has a definition (value). Dictionaries are: Unordered (in Python versions before 3.7) Ordered (from Python 3.7 onwards) Mutable (can be changed) Indexed by keys, not positions