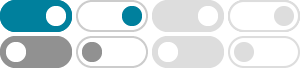
python - Adding dictionaries together - Stack Overflow
The easiest way to do it is to simply use your example code, but using the items() member of each dictionary. So, the code would be: dic0 = {'dic0': 0} dic1 = {'dic1': 1} dic2 = dict(dic0.items() + dic1.items())
python - How do I merge a list of dicts into a single dict? - Stack ...
def merge_dicts(list_of_dicts): unique_kv_pairs = set((k, v) for d in list_of_dicts for k, v in d.items()) merged_dict = dict(unique_kv_pairs) assert len(unique_kv_pairs) == len(merged_dict), "dict merge conflict" return merged_dict
How do I merge two dictionaries in a single expression in Python?
How can I merge two Python dictionaries in a single expression? For dictionaries x and y, their shallowly-merged dictionary z takes values from y, replacing those from x. In Python 3.9.0 or greater (released 17 October 2020, PEP-584, discussed here): z = x | y In Python 3.5 or greater: z = {**x, **y} In Python 2, (or 3.4 or lower) write a function:
Merging or Concatenating two Dictionaries in Python
Jan 23, 2025 · update () method can be used to merge dictionaries. It modifies one dictionary by adding or updating key-value pairs from another. Explanation: d1.update (d2) adds all key-value pairs from d2 to d1. If a key exists in both d1 and d2 (e.g., ‘y‘), …
Python | Concatenate dictionary value lists - GeeksforGeeks
Apr 27, 2023 · In this example, We’ve used the reduce () function to iterate over the values of the dictionary and to concatenate them together. The lambda function defines how the lists are being concatenated together.
Dictionary Add/Append Programs - GeeksforGeeks
Feb 12, 2025 · In this guide, we’ll cover a variety of dictionary add/append operations, from simple key-value insertion to handling nested structures, user input, and list-based dictionaries. We’ll also explore how to concatenate dictionary values, append new data efficiently, and work with tuples as dictionary keys.
Python Merge Dictionaries - Combine Dictionaries (7 Ways)
Nov 18, 2021 · In this tutorial, you’ll learn how to use Python to merge dictionaries. You’ll learn how to combine dictionaries using different operators, as well as how to work with dictionaries that contain the same keys. You’ll also learn how to append list values when merging dictionaries. Python dictionaries are incredibly important data structures to learn.
How to Concat Dict in Python? - Python Guides
Sep 30, 2024 · Discover various methods to concatenate dictionaries in Python, including the update() method, | operator, dictionary unpacking, and more, with detailed examples and syntax
Python Dictionary: Guide To Work With Dictionaries In Python
What is a Python Dictionary? A Python dictionary is a collection of key-value pairs where each key must be unique. Think of it like a real-world dictionary where each word (key) has a definition (value). Dictionaries are: Unordered (in Python versions before 3.7) Ordered (from Python 3.7 onwards) Mutable (can be changed) Indexed by keys, not ...
How to Easily Merge Dictionaries in Python | Newtum
Jan 29, 2025 · Learn how to merge dictionaries in Python using update(), unpacking (**), and dictionary comprehension with practical examples.