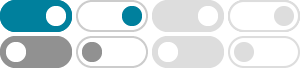
Display a countdown for the python sleep function
You can do a countdown function like: import sys import time def countdown(t, step=1, msg='sleeping'): # in seconds pad_str = ' ' * len('%d' % step) for i in range(t, 0, -step): print '%s for the next %d seconds %s\r' % (msg, i, pad_str), sys.stdout.flush() time.sleep(step) print 'Done %s for %d seconds!
How To Create a Countdown Timer Using Python? - GeeksforGeeks
May 17, 2022 · In this project, we will be using the time module and its sleep () function. Follow the below steps to create a countdown timer: Step 1: Import the time module. Step 2: Then ask the user to input the length of the countdown in seconds. Step 3: This value is sent as a parameter ‘t’ to the user-defined function countdown ().
Python sleep(): How to Add Time Delays to Your Code
You'll use decorators and the built-in time module to add Python sleep() calls to your code. Then, you'll discover how time delays work with threads, asynchronous functions, and graphical user interfaces.
The Definitive Guide to time.sleep() in Python – TheLinuxCode
Dec 22, 2024 · By combining time.sleep() with a loop, we can construct a basic terminal-based countdown timer. Here is an example that counts down 5 seconds: import time seconds = 5 while seconds > 0: print(seconds) time.sleep(1) seconds -= 1 print("Liftoff!")
How to Create a Countdown Timer in Python - Delft Stack
Feb 2, 2024 · This tutorial introduces how to create a countdown timer in Python. The code accepts an input of how long the countdown should be and will start the countdown immediately after the input is entered. Using the time Module and the …
How to Make Precise Time Delays in Python with sleep()
By sleeping 250 ms between each frame, we craft a smooth 1200 ms countdown before "liftoff!". This level of precision opens up new possibilities like games, timers, progress bars, typing simulations, and much more! Next let‘s look at how to generate dynamic time delays…
How To Create A Countdown In Python - CodeSpeedy
In this tutorial, we will see how to create a countdown in python. The code will take input from the user regarding the length of the countdown in seconds. After that, a countdown will begin on the screen of the format ‘minutes:seconds’. We will be using the time module for this program. time.sleep() We will be using the time module.
Mastering `time.sleep()` in Python - CodeRivers
Jan 23, 2025 · Whether you're creating a simple countdown timer, implementing a delay between network requests to avoid overloading a server, or adding a smooth animation effect in a graphical application, `time.sleep()` can be your go-to function.
Python time sleep () Method - Learn By Example
Learn about Python's time.sleep() method: its usage, syntax, parameters, working, and examples including adding a delay, creating a countdown timer, simulating loading/progress, polling with timeout, and rate limiting requests.
Python sleep() (With Examples) - Programiz
The sleep() method suspends the execution of the program for a specified number of seconds. In the tutorial, we will learn about the sleep() method with the help of examples. Learn to code solving problems and writing code with our hands-on Python course.