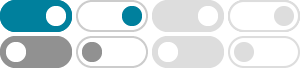
Implement Stack using Array - GeeksforGeeks
Mar 21, 2025 · To implement a stack using an array, initialize an array and treat its end as the stack’s top. Implement push (add to end), pop (remove from end), and peek (check end) operations, handling cases for an empty or full stack. Step-by-step approach: Initialize an array to represent the stack. Use the end of the array to represent the top of the stack.
How to Implement Stack in Java Using Array and Generics?
Feb 14, 2023 · There are 4 primary operations in the stack as follows: push () Method adds element x to the stack. pop () Method removes the last element of the stack. top () Method returns the last element of the stack. empty () Method returns whether the stack is empty or not. Note: Time Complexity is of order 1 for all operations of the stack. Illustration:
Stack Implementation Using Array in Java - Java Guides
The operations that can be performed on a stack are: push (x): Adds an element x to the top of the stack. pop (): Removes and returns the top element of the stack. peek (): Returns the top element without removing it. isEmpty (): Checks if the stack is empty.
Java Stack Implementation using Array - HowToDoInJava
Mar 31, 2023 · This tutorial gives an example of implementing a Stack data structure using an Array. The stack offers to put new objects on the stack (push) and to get objects from the stack (pop). A stack returns the object according to last-in-first-out (LIFO). Please note that JDK provides a default java stack implementation as class java.util.Stack.
Java Program to Implement stack data structure
Java provides a built Stack class that can be used to implement a stack. import java.util.Stack; class Main { public static void main(String[] args) { // create an object of Stack class Stack<String> animals= new Stack<>(); // push elements to top of stack animals.push("Dog"); animals.push("Horse"); animals.push("Cat"); System.out.println ...
Implement Stack in Java Using Array and Generics - Online …
Jul 27, 2023 · The stack class uses an array of type T[] to store elements and keeps track of the topmost element using an integer variable called "top". It provides essential methods like push, pop, peek, and isEmpty, ensuring efficient stack operations.
10 Ways to Master Stack Implementation in Java using Arrays: A ...
Jun 27, 2024 · Array-based stacks in Java use an array and an index (“top”) to keep track of elements, with push operations adding to the end of the array and pop operations removing from the end. Error handling is crucial; you must address stack overflow (when the array is full) and stack underflow (when attempting to pop from an empty array).
Stack Implementation using Array in Java - JavaByTechie
Jan 19, 2025 · In this tutorial page, we are going to learn about how to implement a stack data structure using an array in Java. By the end of this tutorial, you will have a clear understanding of both stack operations and how to effectively utilize an Array in Java.
Stack Implementation using Array in Java - Daily Java Concept
Apr 20, 2020 · Class 2 ie; StackArrayImplementation.java has implemented StackArray.java and provide the implementation for Stack using Array. This Java class defines a simple stack implementation using an array. Let’s break down the key components and methods: Initializes the stack with the specified size.
Create or implement stack using array in java (with example)
Oct 8, 2016 · Given a array of integers, implement stack using array in java (with example). Create push & pop operations of stack to insert & delete element.