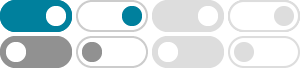
Swap three numbers in cycle - GeeksforGeeks
Apr 20, 2023 · Given three numbers, swap them in cyclic form. First number should get the value of third, second should get the value of first and third should get value of second. Examples:
Swap three variables without using temporary variable
Nov 4, 2021 · The bitwise XOR operator can be used to swap three variables. The idea is similar to method 1. We first store XOR of all numbers in ‘a’. Then we get individual numbers by doing XOR of this with other two numbers.
JavaScript: Swap three numbers - w3resource
Mar 1, 2025 · Write a JavaScript program to swap three numbers without a third variable. * Swaps the values of three variables using arithmetic operations. * @param {number} x - The first number. * @param {number} y - The second number. * @param {number} z - The third number. * @returns {Array} - An array containing the swapped values of x, y, and z. */
Javascript Number Swapping - Stack Overflow
Jul 9, 2021 · You can use truncate to remove decimals and then covert to string, reverse the string and convert back to number. function swapNumber(num) { let truncatedNum = Math.trunc(num) if (num > 9 && num < 100) { return Number((truncatedNum.toString()).split("").reverse().join("")) } else { return "Input 2 …
How to swap two variables in JavaScript - Stack Overflow
Apr 24, 2013 · Till ES5, to swap two numbers, you have to create a temp variable and then swap it. But in ES6, its very easy to swap two numbers using array destructuring. See example. let x,y; [x,y]=[2,3]; console.log(x,y); // return 2,3 [x,y]=[y,x]; console.log(x,y); // return 3,2
4 Ways to Swap Variables in JavaScript - Dmitri Pavlutin Blog
Mar 23, 2023 · JavaScript offers a bunch of good ways to swap variables, with and without additional memory. The first way, which I recommend for general use, is swapping variables by applying destructuring assignment [a, b] = [b, a] .
10 Ways To Swap Values In JavaScript - codeburst
Aug 11, 2020 · We can use some mathematics magic to swap your values. Whaaat!? Yep, so let’s see how it’s working. We get the sum of both numbers on line 4. Now, if we subtract one number from the sum then we get the other number right. That’s what we …
JavaScript Program to Swap Two Variables
In this example, you will learn to write a program to swap two variables in JavaScript using various methods.
Javascript program to swap two numbers without using
May 28, 2024 · Below are the approaches used to swap two numbers without using a temporary variable: Example 1: The idea is to get a sum in one of the two given numbers. The numbers can then be swapped using the sum and subtraction from the sum. Example 2: Multiplication and division can also be used for swapping.
javascript - using for loops to swap digits - Stack Overflow
Apr 9, 2024 · using a for loop i have to write code to swap the 2nd and 3rd digit of a number given via user input in javascript. if (i === 0) num2 = entNum[i]; else if (i === entNum.length - 2) { newNum = newNum + num2; newNum = entNum[i] + newNum; else newNum = …
- Some results have been removed