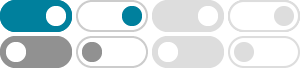
Shell Sort Algorithm in C | Shell Sort Program in C - Sanfoundry
Write a C program to sort a given array of integer numbers using Shell Sort algorithm.
C Exercises: Sort numbers using shell sorting method
Mar 20, 2025 · Write a C program to implement shell sort on an array of floating-point numbers and verify the sorted order. Write a C program to optimize shell sort using the Sedgewick gap sequence and count comparisons.
Shell Sort - GeeksforGeeks
Feb 20, 2025 · In Shell sort, we make the array h-sorted for a large value of h. We keep reducing the value of h until it becomes 1. An array is said to be h-sorted if all sublists of every h’th element are sorted. Algorithm: Step 2 − Initialize the value of gap size, say h. Step 3 − Divide the list into smaller sub-part. Each must have equal intervals to h.
Shell Sort (With Code in Python, C++, Java and C) - Programiz
Shell sort is an algorithm that first sorts the elements far apart from each other and successively reduces the interval between the elements to be compared. In this tutorial, you will understand the working of shell sort with working code in C, C++, Java, and Python.
C Program for Sorting an Array using Shell Sort - CodezClub
Sep 15, 2017 · Write a C Program for Sorting an Array using Shell Sort. Here’s simple C Program for Sorting an Array using Shell Sort in C Programming Language. Shell Sort is mainly a variation of Insertion Sort.
C Program to Implement SHELL SORT
#include<stdio.h> void ShellSort(int a[], int n) { int i, j, increment, tmp; for(increment = n/2; increment > 0; increment /= 2) { for(i = increment; i < n; i++) { tmp = a[i]; for(j = i; j >= increment; j -= increment) { if(tmp < a[j-increment]) a[j] = a[j-increment]; else break; } a[j] = tmp; } } } int main() { int i, n, a[10]; printf("Enter ...
Shell Sort Algorithm Implementation in C: Knuth’s and Hibbard’s ...
Feb 7, 2025 · Shell Sort Algorithm sorts elements in array at a specific interval. At first, it sorts the elements that are away from each other and successively reduces the interval between them. This is a simple but powerful version of another sorting algorithm insertion sort.
Implementation of Shell Sort Algorithm in C - Simple2Code
Jan 20, 2022 · In this tutorial, we will write a C program to implement shell sort. Before that, you may go through the following topics in C. Shell sort is an in-place comparison-based sorting algorithm and variation of Insertion sort. It is a better version of …
Shell Sort Program in C - Online Tutorials Library
Shell Sort Program in C - Learn how to implement the Shell Sort algorithm in C with our easy-to-follow example. Explore the code and understand its functionality.
C Program to Perform Shell Sort without using Recursion
* C Program to Perform Shell Sort without using Recursion */ #include <stdio.h> #define size 7 /* Function Prototype */ int shell_sort (int []); void main {int arr [size], i; printf ("Enter the elements to be sorted:"); for (i = 0; i < size; i ++) {scanf ("%d", & arr [i]);} shell_sort (arr); printf ("The array after sorting is:"); for (i = 0; i ...
- Some results have been removed