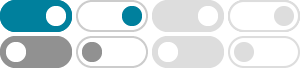
Java Program to Swap two Variables - GeeksforGeeks
Jul 2, 2024 · Java Program to Swap Two Numbers Problem Statement: Given two integers m and n. The goal is simply to swap their values in the memory block and write the java code demonstrating approaches.
Java Program to Swap Two Numbers - GeeksforGeeks
Sep 30, 2024 · Problem Statement: Given two integers m and n. The goal is simply to swap their values in the memory block and write the java code demonstrating approaches. Illustration: Approaches: There are 3 standard approaches to swap numbers varying from space and time complexity. Creating an auxiliary memory cell in the memory.
Java Program to Swap Two Numbers
In this program, you'll learn two techniques to swap two numbers in Java. The first one uses a temporary variable for swapping, while the second one doesn't use any temporary variables.
How to write a basic swap function in Java - Stack Overflow
Here's a method to swap two variables in java in just one line using bitwise XOR(^) operator. class Swap { public static void main (String[] args) { int x = 5, y = 10; x = x ^ y ^ (y = x); System.out.println("New values of x and y are "+ x + ", " + y); } }
Java: Swap two variables - w3resource
Apr 1, 2025 · Write a Java program to swap two variables. Java: Swapping two variables. Swapping two variables refers to mutually exchanging the values of the variables. Generally, this is done with the data in memory. The simplest method to swap two variables is to use a third temporary variable : define swap(a, b) temp := a a := b b := temp
Swap Two Variables in Java - Baeldung
Feb 14, 2025 · In this article, we looked at how to swap two variables in Java, depending on the type of the variables. We described how to swap Objects, and then we studied several ways to swap primitives types with several algorithms. Finally, we had a …
Is it possible to swap two variables in Java? - Stack Overflow
May 1, 2012 · Given two values x and y, I want to pass them into another function, swap their value and view the result. Is this possible in Java? "Swap their value"? Do you mean variables x and y? And you want the two variables to have each other's value after the call? Have you checked this Program to Swap two variables or number.
java - swap two numbers using call by reference - Stack Overflow
Feb 20, 2012 · Java does not have "call by reference". Yes and no. Java never passes by reference, and your way is one workaround. But yet you create a class just to swap two integers. Instead, you can create an int wrapper and use pass it, this way the integer may be separated when not needed: public int value; int temp = a.value; a.value = b.value;
Mastering Variable Swapping in Java: A Comprehensive Guide
This tutorial will delve deep into various methods to swap two variables in Java, catering to both beginners and experienced developers. We will explore basic techniques, as well as some advanced approaches like using bitwise operations.
Swap Two Numbers in Java - Sanfoundry
Here is a Java program that will swap two numbers using a temporary variable or without using a temporary variable, along with examples.