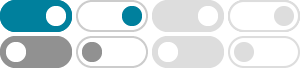
algorithm - creating a spiral array in python? - Stack Overflow
Apr 25, 2016 · if x == y or (x < 0 and x == -y) or (x > 0 and x == 1 - y): dx, dy = -dy, dx x, y = x + dx, y + dy spiral_matrix_size = 5 my_list = list(range(spiral_matrix_size**2)) my_list = [my_list[x:x + spiral_matrix_size] for x in range(0, len(my_list), spiral_matrix_size)] print(my_list) for i, (x, y) in enumerate(spiral(spiral_matrix_size, spiral ...
How to format a given array (matrix) in spiral form ... - Our Code …
Nov 28, 2018 · return all elements of the matrix in spiral order. [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] It should return [1,2,3,6,9,8,7,4,5]. res = [] if len(matrix) == 0: return res. row_begin = 0. row_end = len(matrix) - 1. col_begin = 0. col_end = len(matrix[0]) - 1. while row_begin <= row_end and col_begin <= col_end: for i in range(col_begin, col_end+1):
Print a given matrix in spiral form - GeeksforGeeks
Dec 21, 2024 · Given a matrix mat [] [] of size m x n, the task is to print all elements of the matrix in spiral form. Examples: The idea is to simulate the spiral traversal by marking the cells that have already been visited.
Python: How to arrange number sequence in a spiral
Sep 8, 2022 · I want to write a function that takes a number (n) and outputs a sequence of numbers (1 to n x n) in the form of an n*n grid, with the numbers arranged in a spiral. The starting position is either the center.
How do I make make spiral of natural numbers in python?
Jan 10, 2023 · I want to make a function that I give it a number and the function returns a spiral from 1 to that number(in 2 dimensional array). For example if I give the number 25 to the function it will return something like this:
python - Making an array of spiral numbers - Code Review Stack Exchange
Oct 24, 2020 · for i in range(selected_num_pos+1, n): if array_dup[selected_row][i] == -1: its_neg = True. break. array[selected_row][i] = count. array_dup[selected_row][i] = -1. count += 1. if its_neg: selected_num_pos = i-1. else: selected_num_pos = i. its_neg = False. for i in range(selected_row+1, n): if array_dup[i][selected_num_pos] == -1: its_neg = True.
Spiral Matrix Solution in Python
Given the following matrix: [1, 2, 3], [4, 5, 6], [7, 8, 9] You should return [1, 2, 3, 6, 9, 8, 7, 4, 5]. It’s like a delicious spiral of numbers! def spiralOrder(self, matrix: list[list[int]]) -> list[int]: if not matrix: return [] m = len(matrix) n = len(matrix[0]) ans = [] r1 = 0. c1 = 0. r2 = m - 1. c2 = n - 1.
Spiral Matrix - LeetCode
Given an m x n matrix, return all elements of the matrix in spiral order. Example 1: Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [1,2,3,6,9,8,7,4,5] Example 2: Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] Output: [1,2,3,4,8,12,11,10,9,5,6,7] Constraints: m == matrix.length; n == matrix[i].length; 1 <= m, n <= 10-100 <= matrix[i][j ...
Implementing the Merge Sort Algorithm in Python - Codecademy
Mar 24, 2025 · Whether it’s organizing a to-do list, ranking search results, or arranging numbers in order, efficient sorting is important for handling large amounts of data. In this tutorial, we will explore how to implement merge sort in Python, a powerful sorting algorithm that uses a divide-and-conquer approach.
Spiral Matrix in Python - devscall.com
Jul 21, 2024 · Solve the Spiral Matrix problem in Python. Learn algorithms to traverse a matrix in a spiral order with Python code and detailed explanations for optimal solutions.
- Some results have been removed