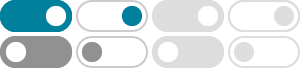
c - Sorting a Stack in Ascending Order? - Stack Overflow
Jul 8, 2012 · Push_swap: sorting a given set of numbers in ascending order in C, using two rotatable stacks with limited instructions
Sort a stack using a temporary stack - GeeksforGeeks
Mar 17, 2025 · Given a stack of integers, sort it in ascending order using another temporary stack. Examples: To sort a stack of intergers using an extra stack (tmpStack), first, create an empty tmpStack. Then, while the input stack is not empty, pop the top element (temp). If tmpStack has smaller elements on top, move them back to the input stack.
C Program To Arrange Numbers in Ascending Order
In this post, we will learn how to arrange numbers in ascending order using C Programming language. This program asks the user to enter n elements, then this program sorts and arrange the entered numbers in the ascending order.
c - How to sort a stack using only stack operations ... - Stack Overflow
Sep 10, 2016 · Given a stack S, write a C program to sort the stack (in the ascending order). We are not allowed to make any assumptions about how the stack is implemented. The only functions to be used are:
c - Simpler way of sorting three numbers - Stack Overflow
I did this using the brute force method; Write a program that receives three integers as input and outputs the numbers in increasing order. Do not use loop / array.
Sorting array using Stacks - GeeksforGeeks
Aug 12, 2022 · We basically use Sort a stack using a temporary stack. Then we put sorted stack elements back to the array. Implementation: Complexity Analysis: Time Complexity: O (n*n). Auxiliary Space: O (n) since auxiliary array is being used to create stack.
C Program to Sort an Array in Ascending Order - GeeksforGeeks
Nov 20, 2024 · Sorting an array in ascending order means arranging the elements in the order from smallest element to largest element. The easiest way to sort an array in C is by using qsort() function. This function needs a comparator to know how to compare the values of the array.
C Program to Sort an Array in Ascending Order - Sanfoundry
Here is source code of the C program to sort the array in an ascending order. The program is successfully compiled and tested using Turbo C compiler in windows environment. The program output is also shown below. * C program to accept N numbers and arrange them in an ascending order. */ a = number [i]; number [i] = number [j]; number [j] = a;
C Exercises: Sort a stack using another stack - w3resource
Mar 20, 2025 · Write a C program to sort a given stack using another stack. Sample Solution: if (top == MAX_SIZE - 1) { printf("Overflow stack!\n"); return; top++; stack[top] = data; if (top == -1) { printf("Empty Stack!\n"); return -1; int data = stack[top]; top--; return data; int temp; int sortedTop = -1; // Top of the stack used for sorting. // Sorting logic.
C Program to arrange numbers in ascending order
Feb 9, 2015 · The following program prompts user for the n numbers, once the user is done entering those numbers, this program sorts and displays them in ascending order. Here we have created a user defined function sort_numbers_ascending() for the sorting purpose.
- Some results have been removed